نحوه خودکارسازی ایجاد کاربران و گروه ها در لینوکس با استفاده از Bash Script.
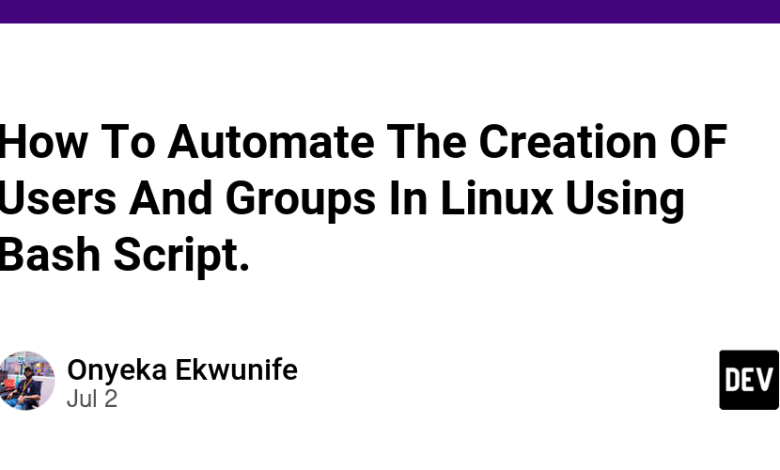
Summarize this content to 400 words in Persian Lang
معرفیتصور کنید در یک شرکت بسیار بزرگ کار می کنید، و شرکت شما حدود 100 کارمند جدید استخدام کرده است و مسئولیت ایجاد حساب های کاربری برای آنها و همچنین اضافه کردن آنها به گروه های مختلف در یک سیستم لینوکس بر عهده شماست.
انجام این کارها به صورت دستی می تواند بسیار خسته کننده و همچنین مستعد خطا باشد. در این پست، من شما را با فرآیند خودکارسازی این فرآیند با استفاده از یک اسکریپت ساده BASH آشنا خواهم کرد.
الزامات
ماشین لینوکس
دانش اولیه اسکریپت نویسی
یک فایل txt که حاوی نام کارمندان (کاربران) و گروههای آنها است N/B: نامهای کاربری و گروهها باید با «;» از هم جدا شوند و در شرایطی که کاربر به بیش از یک گروه تعلق دارد، گروهها باید با کاما (‘,’) از هم جدا می شوند. نمونه زیر را بررسی کنید؛
staff.txt
Onyeka;electronics,devOps
Charles;admin
Bukola;marketing
وارد حالت تمام صفحه شوید
از حالت تمام صفحه خارج شوید
مرحله 1ترمینال خود را باز کنید و یک اسکریپت به نام create_users.sh ایجاد کنید، می توانید از nano یا vim استفاده کنید
nano create_users.sh
گام 2بیایید دایرکتوری هایی برای ذخیره کاربران تولید شده و رمز عبور آنها و همچنین فایل های گزارش ایجاد کنیم. ما مطمئن می شویم که shebang (#!/bin/bash) قبل از هر چیز دیگری در بالای اسکریپت اضافه شده است.
#!/bin/bash
#create main directory to save files
mkdir var
cd var #move inside the created dir
#create log folder and user_mgt.log inside the folder
mkdir log && touch log/user_management.log
#create secure folder and user_passwd file inside the folder
mkdir secure && touch secure/user_passwords.txt
#Read and Write permission for the owner only
chmod 700 secure
# go back to the home dir
cd ..
وارد حالت تمام صفحه شوید
از حالت تمام صفحه خارج شوید
همانطور که در بالا نشان داده شد، اسکریپت یک dir به نام var ایجاد می کند، در داخل var dir، دو پوشه دیگر با نام های log و امن با user_management.log و user_passwords.txt در داخل آنها ایجاد می شود. سپس با استفاده از #chmod دسترسی به پوشه امن را محدود کنید.
مرحله 3در اینجا، ما توابعی برای ایجاد رمز عبور تصادفی، ایجاد کاربر جدید، گروه جدید و افزودن کاربران ایجاد شده به گروههای مختلف ایجاد میکنیم.
#function to generate password
generate_password() {
local password=$(openssl rand -base64 12)
echo “$password”
}
#Create users, groups and generate password
#for them, then assign groups to the created users
#function to create users
createUser(){
local user=”$1″
id “$user” &>/dev/null
if [ $? -eq 1 ]; then #check if user is existing
sudo useradd -m “$user”
echo “user $user created”
else
echo “$user already created”
fi
}
#function to create group
createGroup(){
local group=”$1″
getent group “$group” &>/dev/null
if [ $? -eq 2 ]; then #check if group has been created
sudo groupadd “$group”
echo “group $group created”
else
echo “$group already created”
fi
}
#function to add users to group
addUser_to_group(){
local user=”$1″
local group=”$2″
sudo usermod -aG “$group” “$user”
echo “$user added to group: $group”
}
وارد حالت تمام صفحه شوید
از حالت تمام صفحه خارج شوید
مرحله 4این نقطه ورود ‘MAIN’ اسکریپت است. ابتدا، از کد زیر برای بررسی آرگومان (فایل txt که شامل کاربران و گروههای آنهاست) برای اهداف اعتبارسنجی ارائه شده استفاده میکنیم، سپس فایل را در یک متغیر (user_file) ذخیره میکنیم.
if [[ $# -ne 1 ]]; then
echo “error: check the file provided”
exit 1
fi
# user details
user_file=”$1″
وارد حالت تمام صفحه شوید
از حالت تمام صفحه خارج شوید
پس از آن، فایل را خط به خط میخوانیم، اعتبار آن را تأیید میکنیم، کاربر ایجاد میکنیم، گروه ایجاد میکنیم و پسوردهایی را برای کاربران ایجاد میکنیم که در قطعه کد زیر نشان داده شده است.
# Check if the file exists
if [[ ! -f “$user_file” ]]; then
echo “user file not found!”
exit 1
fi
# Read the file line by line
while IFS=”;” read -r user groups; do
user=$(echo $user | xargs)
# Check to know if user and group
# contains strings for validation
if [[ -z “$user” && -z “$groups” ]];
then
echo “Empty entry!!”
else
#create group and user if they don’t exist
createUser “$user”
createGroup “$user”
#create group with the same name as the user
sudo usermod -aG “$user” “$user”
#extract the groups one by one
IFS=’,’ read -ra group_array > ./var/secure/user_passwords.txt #PASSWD_PATH
fi
done
وارد حالت تمام صفحه شوید
از حالت تمام صفحه خارج شوید
کد کامل
#!/bin/bash
#create main directory to save files
mkdir var
cd var #move inside the created dir
#create log folder and user_mgt.log inside the folder
mkdir log && touch log/user_management.log
#create secure folder and user_passwd file inside the folder
mkdir secure && touch secure/user_passwords.txt
#Read and Write permission for the owner only
chmod 700 secure
# go back to the home dir
cd ..
#LOG_FILE_PATH=./var/log/user_management.log
#PASSWD_PATH=./var/secure/user_password.txt
#function to generate password
generate_password() {
local password=$(openssl rand -base64 12)
echo “$password”
}
#Create users, groups and generate password
#for them, then assign groups to the created users
#function to create users
createUser(){
local user=”$1″
id “$user” &>/dev/null
if [ $? -eq 1 ]; then #check if user is existing
sudo useradd -m “$user”
echo “user $user created”
else
echo “$user already created”
fi
}
#function to create group
createGroup(){
local group=”$1″
getent group “$group” &>/dev/null
if [ $? -eq 2 ]; then #check if group has been created
sudo groupadd “$group”
echo “group $group created”
else
echo “$group already created”
fi
}
#function to add users to group
addUser_to_group(){
local user=”$1″
local group=”$2″
sudo usermod -aG “$group” “$user”
echo “$user added to group: $group”
}
########## MAIN ENTRY POINT OF THE SCRIPT ##############
#Read and validate .txt file containing
#employees username and groups
# Check if the correct number of arguments is provided
(
if [[ $# -ne 1 ]]; then
echo “error: check the file provided”
exit 1
fi
# user details
user_file=”$1″
# Check if the file exists
if [[ ! -f “$user_file” ]]; then
echo “user file not found!”
exit 1
fi
# Read the file line by line
while IFS=”;” read -r user groups; do
user=$(echo $user | xargs)
# Check to know if user and group
# contains strings for validation
if [[ -z “$user” && -z “$groups” ]];
then
echo “Empty entry!!”
else
#create group and user if they don’t exist
createUser “$user”
createGroup “$user”
#create group with the same name as the user
sudo usermod -aG “$user” “$user”
#extract the groups one by one
IFS=’,’ read -ra group_array > ./var/secure/user_passwords.txt #Log the generated user and password to user_passwords.txt
fi
done
وارد حالت تمام صفحه شوید
از حالت تمام صفحه خارج شوید
در نهایت با اجرای دستور زیر مطمئن شوید که اسکریپت قابل اجرا است.
chmod +x create_users.sh
نحوه استفاده از اسکریپت
./create_users.sh employee.txt #where employee.txt contains user;group(s)
وارد حالت تمام صفحه شوید
از حالت تمام صفحه خارج شوید
این وظیفه کارآموزی HNG من استکارآموزی HNG یک بوت کمپ آنلاین رقابتی برای برنامه نویسان، طراحان و سایر استعدادهای فنی است. این برای افرادی طراحی شده است که می خواهند به سرعت خود را ارتقا دهند، فن آوری های جدید را یاد بگیرند و محصولاتی را در یک محیط مشترک و سرگرم کننده بسازند.https://hng.tech/internshiphttps://hng.tech/premium
معرفی
تصور کنید در یک شرکت بسیار بزرگ کار می کنید، و شرکت شما حدود 100 کارمند جدید استخدام کرده است و مسئولیت ایجاد حساب های کاربری برای آنها و همچنین اضافه کردن آنها به گروه های مختلف در یک سیستم لینوکس بر عهده شماست.
انجام این کارها به صورت دستی می تواند بسیار خسته کننده و همچنین مستعد خطا باشد. در این پست، من شما را با فرآیند خودکارسازی این فرآیند با استفاده از یک اسکریپت ساده BASH آشنا خواهم کرد.
الزامات
- ماشین لینوکس
- دانش اولیه اسکریپت نویسی
- یک فایل txt که حاوی نام کارمندان (کاربران) و گروههای آنها است N/B: نامهای کاربری و گروهها باید با «;» از هم جدا شوند و در شرایطی که کاربر به بیش از یک گروه تعلق دارد، گروهها باید با کاما (‘,’) از هم جدا می شوند. نمونه زیر را بررسی کنید؛
staff.txt
Onyeka;electronics,devOps
Charles;admin
Bukola;marketing
مرحله 1
ترمینال خود را باز کنید و یک اسکریپت به نام create_users.sh ایجاد کنید، می توانید از nano یا vim استفاده کنید
nano create_users.sh
گام 2
بیایید دایرکتوری هایی برای ذخیره کاربران تولید شده و رمز عبور آنها و همچنین فایل های گزارش ایجاد کنیم. ما مطمئن می شویم که shebang (#!/bin/bash) قبل از هر چیز دیگری در بالای اسکریپت اضافه شده است.
#!/bin/bash
#create main directory to save files
mkdir var
cd var #move inside the created dir
#create log folder and user_mgt.log inside the folder
mkdir log && touch log/user_management.log
#create secure folder and user_passwd file inside the folder
mkdir secure && touch secure/user_passwords.txt
#Read and Write permission for the owner only
chmod 700 secure
# go back to the home dir
cd ..
همانطور که در بالا نشان داده شد، اسکریپت یک dir به نام var ایجاد می کند، در داخل var dir، دو پوشه دیگر با نام های log و امن با user_management.log و user_passwords.txt در داخل آنها ایجاد می شود. سپس با استفاده از #chmod دسترسی به پوشه امن را محدود کنید.
مرحله 3
در اینجا، ما توابعی برای ایجاد رمز عبور تصادفی، ایجاد کاربر جدید، گروه جدید و افزودن کاربران ایجاد شده به گروههای مختلف ایجاد میکنیم.
#function to generate password
generate_password() {
local password=$(openssl rand -base64 12)
echo "$password"
}
#Create users, groups and generate password
#for them, then assign groups to the created users
#function to create users
createUser(){
local user="$1"
id "$user" &>/dev/null
if [ $? -eq 1 ]; then #check if user is existing
sudo useradd -m "$user"
echo "user $user created"
else
echo "$user already created"
fi
}
#function to create group
createGroup(){
local group="$1"
getent group "$group" &>/dev/null
if [ $? -eq 2 ]; then #check if group has been created
sudo groupadd "$group"
echo "group $group created"
else
echo "$group already created"
fi
}
#function to add users to group
addUser_to_group(){
local user="$1"
local group="$2"
sudo usermod -aG "$group" "$user"
echo "$user added to group: $group"
}
مرحله 4
این نقطه ورود ‘MAIN’ اسکریپت است. ابتدا، از کد زیر برای بررسی آرگومان (فایل txt که شامل کاربران و گروههای آنهاست) برای اهداف اعتبارسنجی ارائه شده استفاده میکنیم، سپس فایل را در یک متغیر (user_file) ذخیره میکنیم.
if [[ $# -ne 1 ]]; then
echo "error: check the file provided"
exit 1
fi
# user details
user_file="$1"
پس از آن، فایل را خط به خط میخوانیم، اعتبار آن را تأیید میکنیم، کاربر ایجاد میکنیم، گروه ایجاد میکنیم و پسوردهایی را برای کاربران ایجاد میکنیم که در قطعه کد زیر نشان داده شده است.
# Check if the file exists
if [[ ! -f "$user_file" ]]; then
echo "user file not found!"
exit 1
fi
# Read the file line by line
while IFS=";" read -r user groups; do
user=$(echo $user | xargs)
# Check to know if user and group
# contains strings for validation
if [[ -z "$user" && -z "$groups" ]];
then
echo "Empty entry!!"
else
#create group and user if they don't exist
createUser "$user"
createGroup "$user"
#create group with the same name as the user
sudo usermod -aG "$user" "$user"
#extract the groups one by one
IFS=',' read -ra group_array > ./var/secure/user_passwords.txt #PASSWD_PATH
fi
done
کد کامل
#!/bin/bash
#create main directory to save files
mkdir var
cd var #move inside the created dir
#create log folder and user_mgt.log inside the folder
mkdir log && touch log/user_management.log
#create secure folder and user_passwd file inside the folder
mkdir secure && touch secure/user_passwords.txt
#Read and Write permission for the owner only
chmod 700 secure
# go back to the home dir
cd ..
#LOG_FILE_PATH=./var/log/user_management.log
#PASSWD_PATH=./var/secure/user_password.txt
#function to generate password
generate_password() {
local password=$(openssl rand -base64 12)
echo "$password"
}
#Create users, groups and generate password
#for them, then assign groups to the created users
#function to create users
createUser(){
local user="$1"
id "$user" &>/dev/null
if [ $? -eq 1 ]; then #check if user is existing
sudo useradd -m "$user"
echo "user $user created"
else
echo "$user already created"
fi
}
#function to create group
createGroup(){
local group="$1"
getent group "$group" &>/dev/null
if [ $? -eq 2 ]; then #check if group has been created
sudo groupadd "$group"
echo "group $group created"
else
echo "$group already created"
fi
}
#function to add users to group
addUser_to_group(){
local user="$1"
local group="$2"
sudo usermod -aG "$group" "$user"
echo "$user added to group: $group"
}
########## MAIN ENTRY POINT OF THE SCRIPT ##############
#Read and validate .txt file containing
#employees username and groups
# Check if the correct number of arguments is provided
(
if [[ $# -ne 1 ]]; then
echo "error: check the file provided"
exit 1
fi
# user details
user_file="$1"
# Check if the file exists
if [[ ! -f "$user_file" ]]; then
echo "user file not found!"
exit 1
fi
# Read the file line by line
while IFS=";" read -r user groups; do
user=$(echo $user | xargs)
# Check to know if user and group
# contains strings for validation
if [[ -z "$user" && -z "$groups" ]];
then
echo "Empty entry!!"
else
#create group and user if they don't exist
createUser "$user"
createGroup "$user"
#create group with the same name as the user
sudo usermod -aG "$user" "$user"
#extract the groups one by one
IFS=',' read -ra group_array > ./var/secure/user_passwords.txt #Log the generated user and password to user_passwords.txt
fi
done
در نهایت با اجرای دستور زیر مطمئن شوید که اسکریپت قابل اجرا است.
chmod +x create_users.sh
نحوه استفاده از اسکریپت
./create_users.sh employee.txt #where employee.txt contains user;group(s)
این وظیفه کارآموزی HNG من است
کارآموزی HNG یک بوت کمپ آنلاین رقابتی برای برنامه نویسان، طراحان و سایر استعدادهای فنی است. این برای افرادی طراحی شده است که می خواهند به سرعت خود را ارتقا دهند، فن آوری های جدید را یاد بگیرند و محصولاتی را در یک محیط مشترک و سرگرم کننده بسازند.
https://hng.tech/internship
https://hng.tech/premium